New Feature Announcement - Virtual Threads
We're starting off 2024 with a huge announcement!
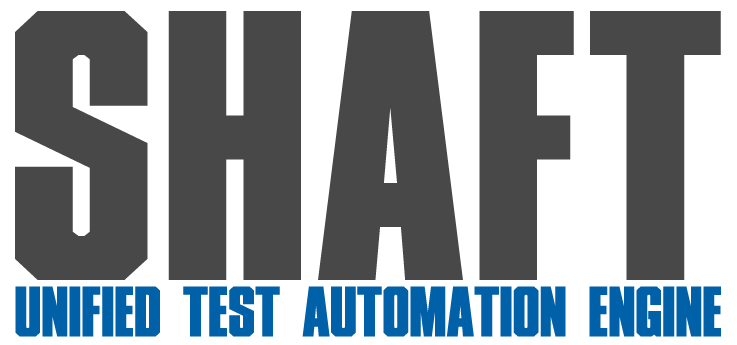
๐ค
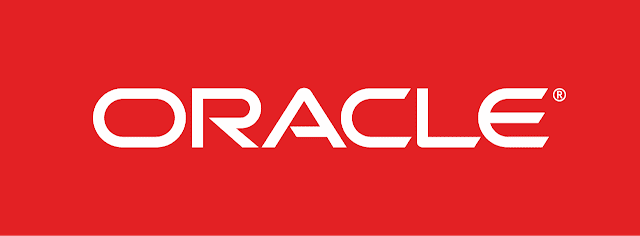
- The future of automation frameworks is here, and it's all about working smarter, not harder.โ
- SHAFT_ENGINE, your trusty automation solution, just got a major upgrade with virtual threads, a clever trick that makes it more efficient and helpful than ever.โ
- What is a Virtual Thread?โ
- Virtual-Threads, introduced with Java 21 are a new way to handle multiple tasks concurrently within a single program or application. They're the new java way for asynchronous operations.
- Think of it like hiring a whole crew of tiny helpers. While one tractor's plowing, another can check the soil, and another can keep an eye on the weather.
- These lightweight assistants don't need fancy equipment or guzzle up resources, meaning SHAFT_ENGINE can now handle a ton more tasks without breaking a sweat.
Now let's think of test automation. In automating a registration form, envision employing a crew of virtual threads as tiny helpers :โ
- Rather than idly waiting for the entire site to load, each virtual thread can be assigned specific tasks as soon as the relevant elements become available. For instance, one thread focuses on inputting the username, another simultaneously handles the email input, and yet another sets the password. This parallel execution optimizes efficiency, ensures prompt responsiveness to dynamically changing elements, and efficiently utilizes resources.
- As the crew of virtual threads collaborates seamlessly, the registration form is filled and submitted swiftly, providing a more agile and responsive automation process compared to traditional sequential approaches.
- Save your execution Time :โ
- That's not all, SHAFT_ENGINE utilities the Virtual Threads for Engine launch and configuration which will make your overall experience a lot faster and swifter.